간단한 WinForms 프로그램
Visual Studio(VS 2010)를 이용해 간단한 윈도우 프로그램을 만드는 과정은 말 그대로 간단하다. VS를 실행한 후, File->New->Project를 선택한다.
새 프로젝트 템플릿 중에서 Windows Forms Application을 선택하면 윈도우 프로그램을 만들 수 있다. 새 윈도우 프로그램은 아래와 같은 폼 하나를 포함한다.
Visual Studio(VS 2010)를 이용해 간단한 윈도우 프로그램을 만드는 과정은 말 그대로 간단하다. VS를 실행한 후, File->New->Project를 선택한다.
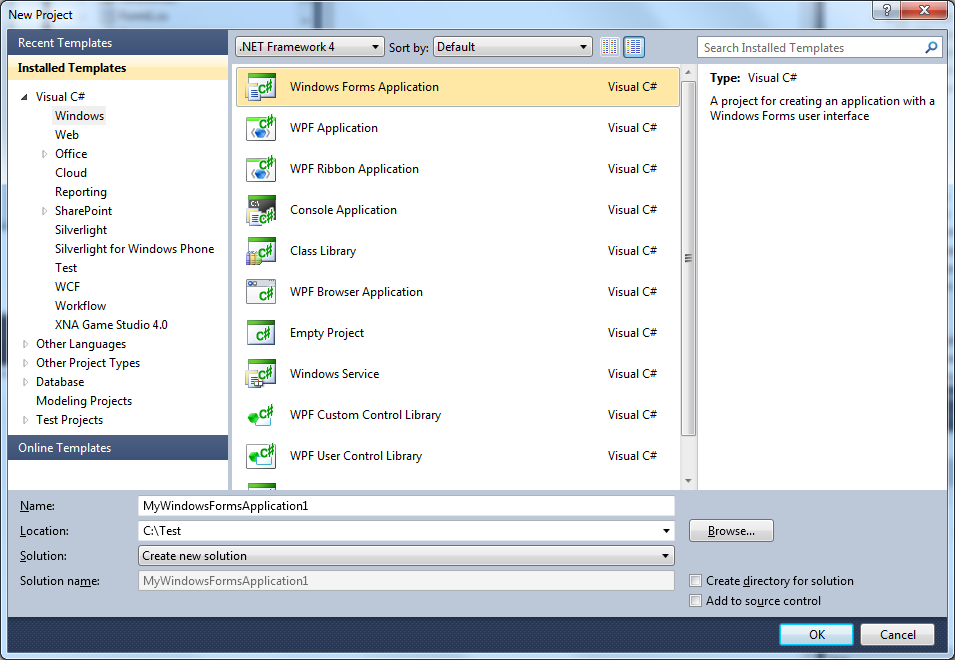
새 프로젝트 템플릿 중에서 Windows Forms Application을 선택하면 윈도우 프로그램을 만들 수 있다. 새 윈도우 프로그램은 아래와 같은 폼 하나를 포함한다.
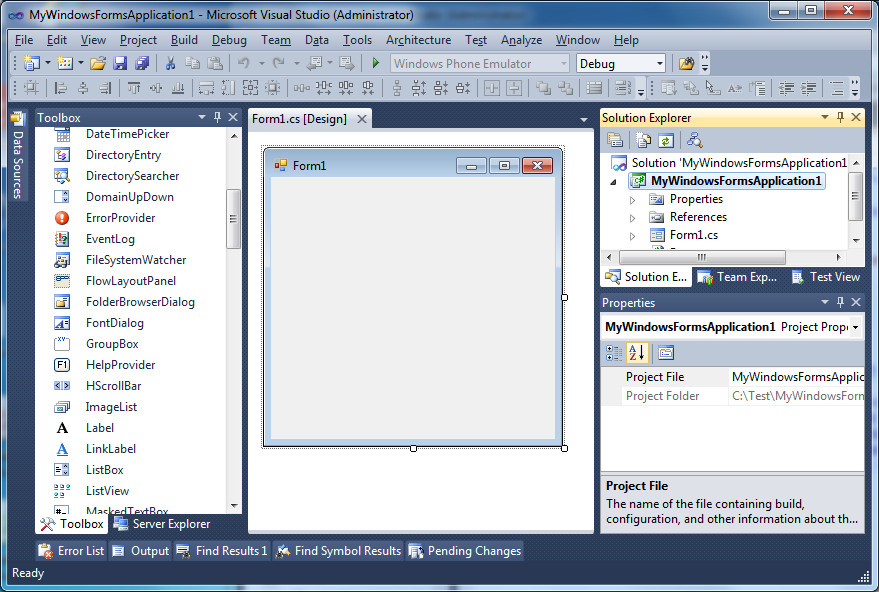
간단한 윈폼의 이해
위의 윈폼 프로그램은 Program.cs와 Form1.cs/Form1.Designer.cs 파일을 생성한다. 우선 프로그램 시작 포인트인 Main()을 살펴 보면, 이 메인에서는 Form1 클래스이 객체를 하나 생성하여, Application.Run()에 파라미터로 넣고 실행한다. Application.Run()은 Form 객체를 화면에 보여주고, 메시지 루프를 만들어 마우스,키보드 등의 입력을 UI (User Interface) 쓰레드에 전달하는 기능을 한다.
예제
using System;
using System.Windows.Forms;
namespace MyWindowsFormsApplication1
{
static class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
}
using System.Windows.Forms;
namespace MyWindowsFormsApplication1
{
static class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
}
- Main() 바로 위의 [STAThread] 해당 메서드가 STA (Single Threaded Arpartment) Thread모델로 실행된다는 것으로 만약 이를 지우면 윈도우 프로그램에 문제가 생기는 경우가 있다.
간단한 윈폼의 이해 2
실제 폼 UI는 Form1.cs과 Form1.Designer.cs 파일에서 정의되는데, Form1.designer.cs는 폼에 포함되는 모든 UI 컨트롤 등에 대한 정보를 가지고 있으며 실행시 이 정보를 기반으로 UI를 그리게 된다. 또한 Visual Studio는 이 파일을 기반으로 폼을 해석(Rendering)해서 VS 디자이너에 보여주게 된다. Form1.cs는 Form1.designer.cs와 동일한 클래스로서 주로 UI의 이벤트를 핸들링 하는 코드들을 적게된다.
예제
// 파일 Form1.cs
using System.Windows.Forms;
namespace MyWindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
}
}
// 파일 Form1.Designer.cs
namespace MyWindowsFormsApplication1
{
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Text = "Form1";
}
#endregion
}
}
using System.Windows.Forms;
namespace MyWindowsFormsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
}
}
// 파일 Form1.Designer.cs
namespace MyWindowsFormsApplication1
{
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.Text = "Form1";
}
#endregion
}
}
- Form1.cs에서 Form1() 즉 클래스 생성자에서 반드시 InitializeComponent()를 호출해야 한다. 이는 UI 컴포넌트를 생성하는 역활을 한다.
- Form1.cs 와 Form1.Designer.cs는 같은 클래스로서 2개 파일로 나뉘어진 경우다. 이것을 C#에서 Partial Class라고 부른다.
C# 동영상 강의 : C# 첫 윈폼 프로그램 만들기 [레벨] 초급 ![]() [C# 기초] 간단한 예제를 들어 윈폼을 만드는 과정과 Visual Studio 의 관련 기능들, 그리고 폼이 생성되었을 때의 폼 관련 파일들에 대해 설명합니다. |